Parallel Arrays As part of ‘Teach for America’, you have been recruited to teach in a remote Appalachian community.
Parallel Arrays As part of ‘Teach for America’, you have been recruited to teach in a remote Appalachian community.
April 11, 2021 Comments Off on Parallel Arrays As part of ‘Teach for America’, you have been recruited to teach in a remote Appalachian community. Assignment Assignment helpParallel Arrays As part of ‘Teach for America’, you have been recruited to teach in a remote Appalachian community. To help analyze students’ performances on an assessment exam, you must write a program that displays each student’s name and then prompts you for that student’s test score. The program will calculate the class average (mean) as well as the deviation of each student′s test score from the mean (the students score minus the average). Finally your program will display the student’s name, his test score, and the deviation from the mean in a readable well-organized format. Currently there are seven students in your class named: Bashful, Doc, Dopey, Grumpy, Happy, Sleepy, and Sneezy. Program Specifications: This program will require you to create 3 parallel arrays, one for the students’ names (data already supplied), one for their test scores (to be read-in from the keyboard), and one for their score′s deviation from the mean (which you will calculate). Create five separate methods to do the following: Display instructions to the user explaining what the program will do and what they are expected to provide in the way of input. Read in the test score from the keyboard and store it into an array. Then return the array to the calling program (this is a value-returning method). Compute the class average (mean) of the test scores and return it to main where it will be later printed to the screen in the display method below. Note this is a value returning method. Compute the deviation from the mean for each student′s score and store it into an array. Then return the array to the calling program where it will be later printed to the screen in the display method below (this is a value-returning method). Display the output in 3 well-aligned columns as presented below. SAMPLE RUN with OUTPUT: This program will read-in test scores for a class of seven students and compute the deviation of each test score from the mean. Each student′s name and test score will be displayed along with the computed deviation from the mean. Please enter student test scores: Enter Doc’s score: 90 Enter Grumpy’s score: 50 Enter Happy’s score: 70 Enter Sleepy’s score: 40 Enter Dopey’s score: 30 Enter Sneezy’s score: 60 Enter Bashful’s score: 80 The average of the scores is 60.0 Name Grade Mean Deviation Doc 90 (30) Grumpy 50 (-10) Happy 70 (10) Sleepy 40 (-20) Dopey 30 (-30) Sneezy 60 (0) Bashful 80 (20) Recommendations for the Simplest Approach: Although there are a variety of ways this problem could be implemented in Java, the most straightforward way to approach it (based on what you have learned thus far) is to create 3 parallel arrays (one array for the student names, one for their scores, and one for the deviations from the mean). A) Set these 3 arrays up in your main method. B) Then declare, instantiate, and initialize the names array with the names of the seven students provided above in the problem desсrіption. C) Create five separate methods to (1) Explain the purpose of the program and provide instructions (2 input the scores for each student, (3) to compute and return the average, (4) to calculate and fill the array for the deviation calculation, and (5) to display the output. Please pass any needed arrays (declared in main) into your methods via the parameter list. (You are not required to set up the output in an actual table format, but just be sure to align columns so that the output is well-organized, aligned, and easily readable.) The java source code for your program (do not forget documentation(comments), both prologue and inline comments.
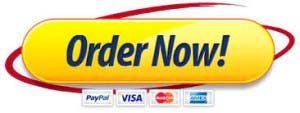
Order Management
Discount
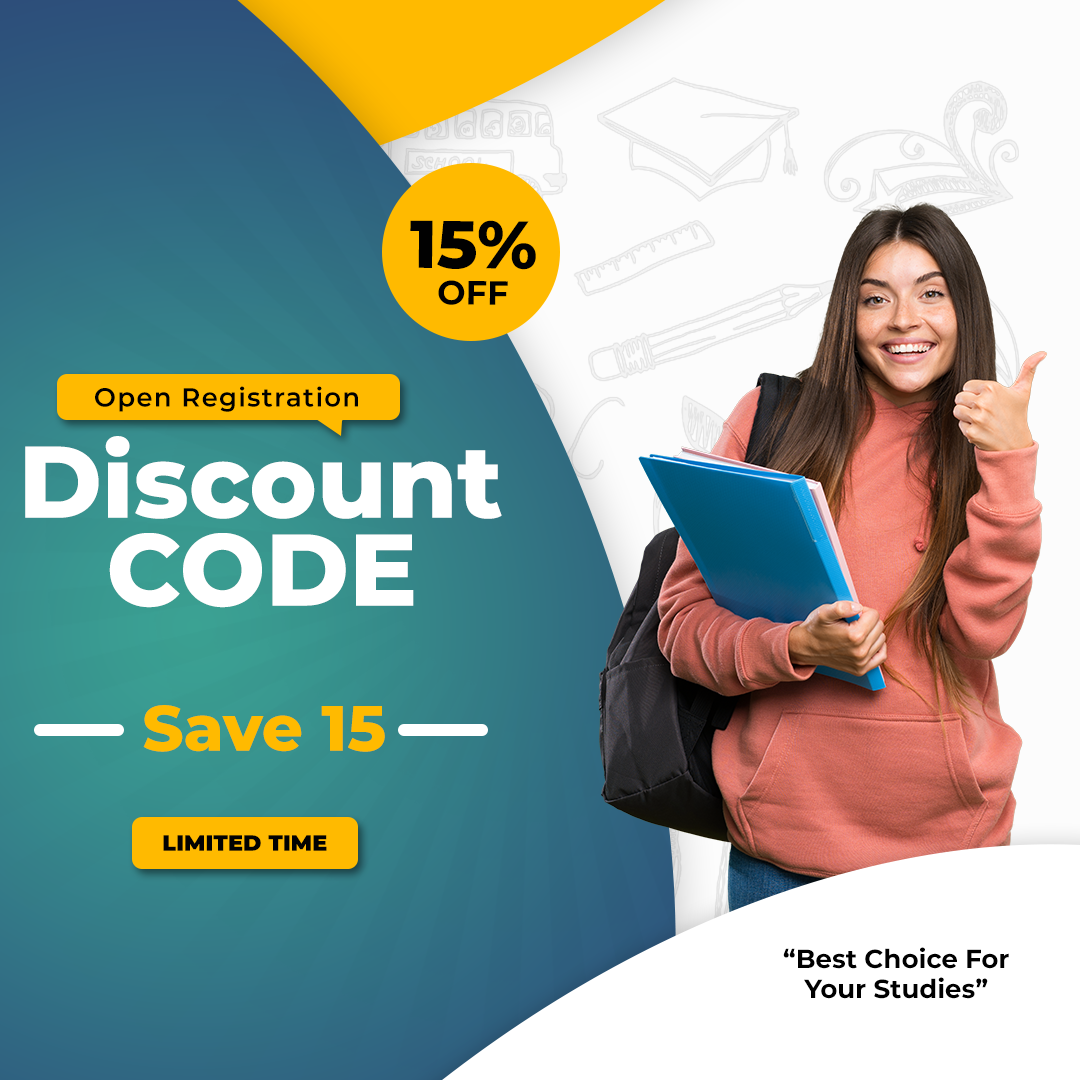
WHY CHOOSE US?
Time-tested quality
Master’s and Ph.D. writers
The expert team of editors
100% plagiarism-free papers
Set of Free features
Talk to your writer directly
Get FREE revision upon request
24/7 Customer Support Department
100% Money-Back guarantees
order now
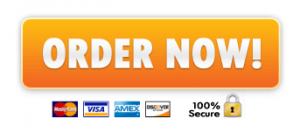
Recent Post
Format and Features
- At least 275 words per page
- Free inquiry
- Free title page
- Free outline
- Free bibliography
- Free plagiarism report
- Free unlimited revisions
- Instant email delivery
- Flexible prices and discounts
Professional academic writer
