Include the functionality that if the user presses the Return key in an entry box the calculation will be performed.
Include the functionality that if the user presses the Return key in an entry box the calculation will be performed.
April 12, 2021 Comments Off on Include the functionality that if the user presses the Return key in an entry box the calculation will be performed. Assignment Assignment helpIntroduction to Software Development has 4 courseworks. Each coursework contributes equally to the overall coursework mark. By doing this coursework you will get experience in developing code in an object oriented style, building a GUI, using libraries (including reading about how to use functionality in a library) . The Exercise – Overview The Questions There are 2 parts. This coursework is marked out of 50. All questions must be answered using Python 3 programming language. Always keep backup copies of all assignments. If your assignment gets lost, a backup copy will make things easier for you. 1.In this assignment you are asked to write a program that maintains a simple database of modules at a University and can answer a number of queries regarding them. Open the Repl term2cw2PartOne, which you can find in on Repl.it. This file has all the following code fragments included. (a)(8 marks) Step One: Module Class First we need a class to model a Module. A module has a name and a code. These are assigned when an object of class module is created. They cannot be altered thereafter. All the methods you need to implement are shown below. class Module: def init (self, name, code): #Creates two instance variables #name is a string #code is a string pass def getCode(self): #Returns the code of the module pass def getName(self): #Returns the name of the module pass def repr (self): #Display the data in the following format #Module Name:then print the name #Code: then the code pass Recall that a code block in Python cannot be empty, so the statement pass has been used as a placeholder (the pass statement does nothing when exectuted). When you are ready to complete a method, remove the pass line. Implement these methods such that the following fragment: m = Module(“Introduction to Software Development”,”BUC71″) print(m) print(“******”) print(m.getName()) print(m.getCode()) Displays: Module Name: Introduction to Software Development Code: BUC71 ****** Introduction to Software Development BUC71 Do NOT have any print statements in your method implementations (and do not hardcode the ” Introduction to Software Development” and BUC71 into your methods). Note that when printing the object, the code is indented by four spaces. Marking Scheme: 2 marks for each of the 4 methods (b)(4 marks) Step Two: Inperson Class Next we construct a class for modules that are taught in-person, called InPerson which inherits from Module For an in-person module we wish to record the additional following information: ˆ Venue class InPerson(Module): # Add the missing implementation to this class # Be sure to call the parent constructor All the additional methods and instance variables are missing from the above class definition. You will need to define and implement these missing methods and an instance variable such that, for the test script: s = InPerson(“Advanced Data Structures”,”BUC22″, “Main Building MAL211”) print(s) print(“******”) print(s.getName()) print(s.getCode()) print(s.getLocation()) The outcome is: Module Name: Advanced Data Structures Code: BUC22 Location: Main Building MAL211 ****** Advanced Data Structures BUC22 Main Building MAL211 (c)(4 marks) Step Three: Online Class Next we construct a class for modules that are taught online, called Online which also inherits from Module For an online module we wish to record the additional following in- formation: ˆ URL class Online(Module): # Add the missing implementation to this class # Be sure to call the parent constructor All the additional methods and instance variables are missing from the above class definition. You will need to define and implement these missing methods and an instance variable such that, for the test script: s = Online(“Programming Paradigms”,”BUC31″, “http:\www.moodle.ac.ukpp.html”) print(s) print(“******”) print(s.getName()) print(s.getCode()) print(s.getLocation()) The outcome is: Module Name: Programming Paradigms Code: BUC31 URL: http:www.moodle.ac.ukpp.html ****** Programming Paradigms BUC31 http:www.moodle.ac.ukpp.html (d)(4 marks) Write a function called DisplayAllDetails displays all the data from a list containing objects of class Online and/or InPerson: For the test script: course = [InPerson(“Advanced Data Structures”,”BUC22″, “Main Building MAL211”), Online(“Programming Paradigms”,"BUC31″,”http:\www.moodle.ac.ukpp.html”), InPerson(“Maths For Computing “,”BUC14”, “Main Building MAL303”), InPerson(“Web Applications”,”BUC54″, “Senate House Room 23”)] DisplayAllDetails(course) The outcome is: Module Name: Advanced Data Structures Code: BUC22 Location: Main Building MAL211 Module Name: Programming Paradigms Code: BUC31 URL: http:www.moodle.ac.ukpp.html Module Name: Maths For Computing Code: BUC14 Location: Main Building MAL303 Module Name: Web Applications Code: BUC54 Location: Senate House Room 23 i.e. the function DisplayAllDetails accepts a list and displays all the data from each element of that list. 2.(20 marks) For the final exercise in this coursework, you are to write a simple GUI for the Lifestage calculator. Open the Repl term2cw2PartTwo, which you can find in on Repl.it. Overview Your program must allow the user to type in a Mammal and an age. Figure 1: LifeStage when started The user can then press the Calculate button. The result is displayed below the buttons: Figure 2: LifeStage after Calculate button clicked Your program should also display the error messages from the life stage calculator from Term2 Coursework 1, see Figure 3. Technical Detail I have provided a skeleton class called LifeStage. Read through this file carefully taking note of my comments. Figure 4 shows what should be displayed when you run this file. Figure 3: Lifestage calculation with user information that causes an error Figure 4: Display from provided code (a)The constructor (7 marks). Add all the widgets that you need to make a display similar to the one shown in Figure 1. I have provided private instance variables that you need to bind to widgets in order to get the information from the entry boxes and to display the answer to the screen. Don’t forget to add a label to display your answer. All widgets are to be displayed using the Grid manager. Ensure the labels and entry widgets are right, left justified respectively. You might want to look at the pady property to put some spacing between widgets. (b)The calculation (7 marks). Use functions from the sample solution for Term2 Coursework 1. Your program will need to read the ap- propriate file (found in the Data folder) containing the data for the chosen mammal and output the result. These functions will need to be turned into methods (i.e.self will need to be the first argument and to call a method you will also need to use self. Hint look at lbs2kgs.py and be sure you follow the logic. (c)Advanced (3 marks). The dynamic display. The reset button is initially set to be disabled. Only when a user types in any entry box does the reset button become enabled. If the reset button is pressed then all the entry boxes and the output label are set to the empty string. The reset button then disables itself. You will need to bind the entry boxes with the “
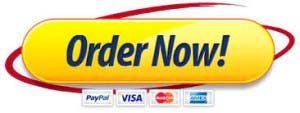
Order Management
Discount
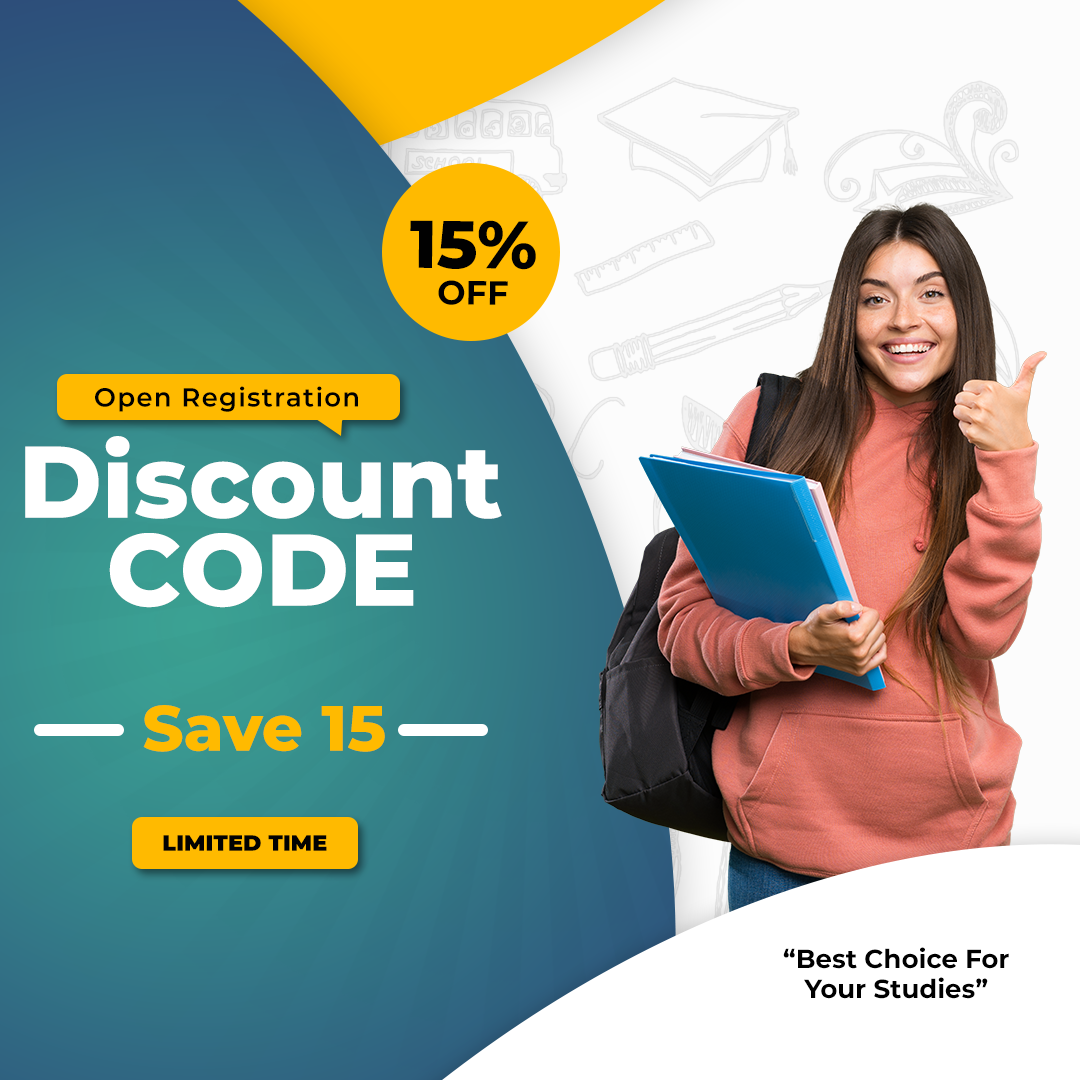
WHY CHOOSE US?
Time-tested quality
Master’s and Ph.D. writers
The expert team of editors
100% plagiarism-free papers
Set of Free features
Talk to your writer directly
Get FREE revision upon request
24/7 Customer Support Department
100% Money-Back guarantees
order now
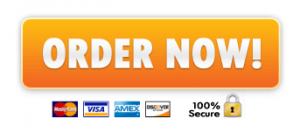
Recent Post
Format and Features
- At least 275 words per page
- Free inquiry
- Free title page
- Free outline
- Free bibliography
- Free plagiarism report
- Free unlimited revisions
- Instant email delivery
- Flexible prices and discounts
Professional academic writer
